In our Daily code life, String is playing a vital role. In this blog, we will discuss taking string value input from users. The user can give any string input. Our goal should be code should work and it should give the desired output.
Scanner class having one method next line(). This method job is taking input string value from the console. Before seeing.
In the previous blog, I posted how to take int value from users. You can check it out.
next() method and nextLine() method is using most of time. we will see first what is next() method and how to use it by one code bit example. One more thing when to use next() and next Line(). we will discuss.
"When you feeling like stopping think about why you started. "
next() method is used for taking input till space. it can't read input separated by space. The next line() method is used for reading input separated by space. this is a main difference between next() and next Line() .
if you want to read only one word you can use the next() method.
more than one word like one sentence or line better to go next Line() method.
Sometimes you don't know what user gives input so my recommendation is to always use the next Line() for the safer side. While interview time, you always write your code by taking user input. it will help to save your time changing input. the interview would be impressed about the way of your writing code.
Let's see the below code you will get to know how to use it in code.
Steps :
// Create Object of Scanner
// Inform the user for input
// hold the input in one string variable
// display the result
Code Bit :
import java.util.Scanner;
public class ExampleScannerInput {
public static void main(String[] args) {
// Create Object of Scanner
Scanner sc = new Scanner(System.in);
// Inform the user for input
System.out.println("Enter a input : ");
// hold the input in one string variable
String input = sc.next();
// display the result
System.out.println("The result is :" + input);
}
}
Screenshot image :
Result :
we saw the next() method code. I have one question if I gave two words separated by space means it will throw an error. Cool yar it will not throw an error. it will print only one word till space. after space, it will not print. That is the next() method specialty. if have curious once try let me know in the comment box. let's go we will see the next Line() method.
Code bit :
import java.util.Scanner;
public class exampleScannerNextLine {
public static void main(String[] args) {
// Create Object of Scanner
Scanner sc = new Scanner(System.in);
// Inform the user for input
System.out.println("Enter a input : ");
// hold the input in one string variable
String input = sc.nextLine();
// display the result
System.out.println("The result is :" + input);
}
}
Screenshot images:
Result :
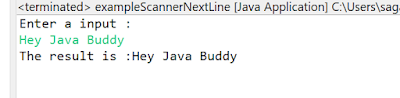
Learned things :
- next line() method: it is used to read user input line or sentence.
- next() method: it is used for reading words until space.
Contribute:
- Regarding Job Details Post
- Referral Jobs Details
- Opening for Freshers
- Knowledge share with us
Send mail to lifeofsrg@gmail.com. will publish with your name and your content.
Learn, practice, and share knowledge
Thank you :)